Create a PM pdf from credit case input
The input to the function CreateDocument is the same kind of case input that is used in most other api-functions in Capitex Företagslån.
The print engine will do a calculation on the case input and then generate the type of documents requested in the call. The document is generated on demand and all data and results is also calculated on demand using the input data.
In this example we request the document called CreditPMPrintout and we want the document in PDF format.
In this case we didn't specify any specific content or templates for the Credit PM so we will get the default template (specified in the configuration of the system, if there is no customer specific credit PM you will get the default built in credit PM template)
Other document formats than PDF that can be used in debug/testing purpose is: text/plain, FO, image/png and HTML.
SOAP Request
This call as all other webservice calls can of course be done from Java using "real" java objects and function calls via a webservice client wrapper like WSDL2Java.
<soapenv:Envelope xmlns:soapenv= "http://schemas.xmlsoap.org/soap/envelope/" xmlns:urn= "urn:types.ApplicationserviceImpl.services.companycalc.capitex" xmlns:xsi= "xsi" > <soapenv:Header/> <soapenv:Body> <urn:CreateDocument> <CreateDocument_1> <InputData> <Companies xsi:type= "sc0:Company" > <ID>30DF1502-08F0-4AEF-A9C2-2C66CC10D056</ID> <ActualFigures xsi:type= "sc0:ActualFigures" > <HistoricalIncomestatements> <Revenue> 100000 </Revenue> </HistoricalIncomestatements> <HistoricalIncomestatements> <Revenue> 200000 </Revenue> </HistoricalIncomestatements> <HistoricalIncomestatements> <Revenue> 300000 </Revenue> </HistoricalIncomestatements> <HistoricalBalanceSheet> <Cash> 200000 </Cash> <UnrestrictedEquity> 200000 </UnrestrictedEquity> </HistoricalBalanceSheet> <HistoricalBalanceSheet> <Cash> 300000 </Cash> <UnrestrictedEquity> 300000 </UnrestrictedEquity> </HistoricalBalanceSheet> <HistoricalBalanceSheet> <Cash> 400000 </Cash> <UnrestrictedEquity> 400000 </UnrestrictedEquity> </HistoricalBalanceSheet> </ActualFigures> </Companies> <CalculationYear> 2020 </CalculationYear> <CalculationMonth> 3 </CalculationMonth> </InputData> <Printouts xsi:type= "sc0:CreditPMPrintout" /> <DocumentFormat>PDF</DocumentFormat> </CreateDocument_1> </urn:CreateDocument> </soapenv:Body> </soapenv:Envelope> |
SOAP Response
<env:Envelope xmlns:ns0= "urn:types.ApplicationserviceImpl.services.companycalc.capitex" xmlns:env= "http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsi= "http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd= "http://www.w3.org/2001/XMLSchema" xmlns:enc= "http://schemas.xmlsoap.org/soap/encoding/" > <env:Body> <ns0:CreateDocumentResponse> <result> <Document>[BASE64-ENCODED-PDF]</Document> <XMLbasedDocument/> </result> </ns0:CreateDocumentResponse> </env:Body> </env:Envelope> |
Page 3, 4 and 5 from the encoded PDF from the response in this example (the other pages is not of interest because the lack of detailed input)
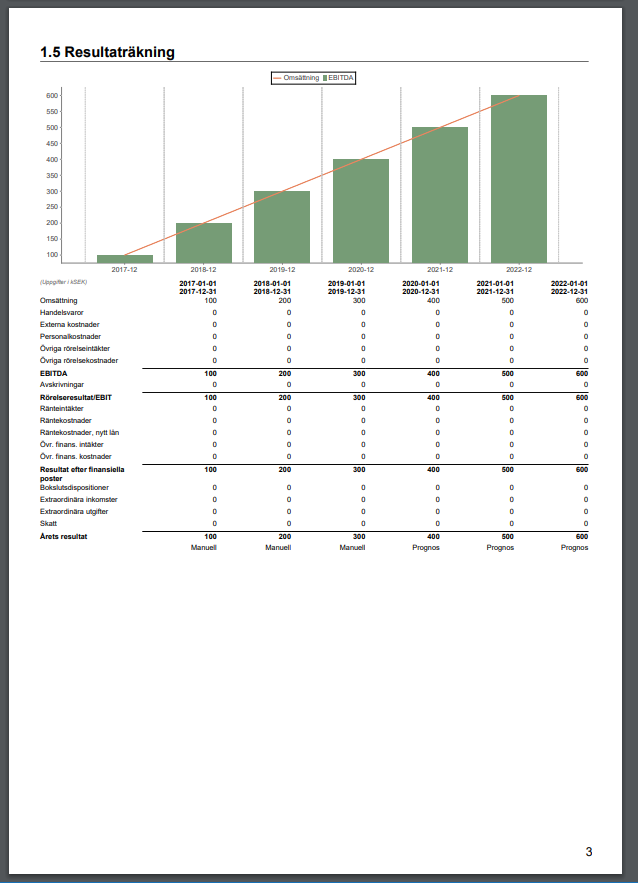
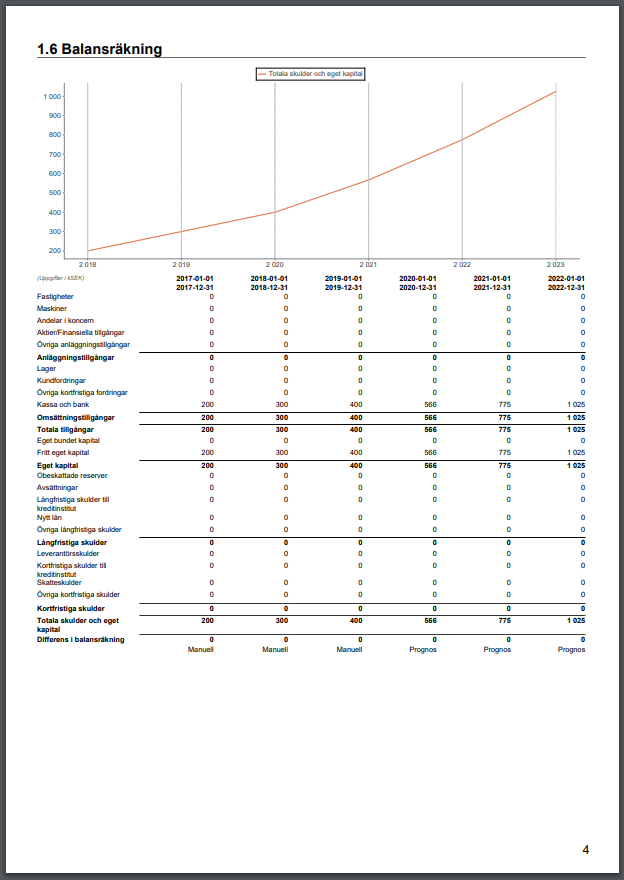
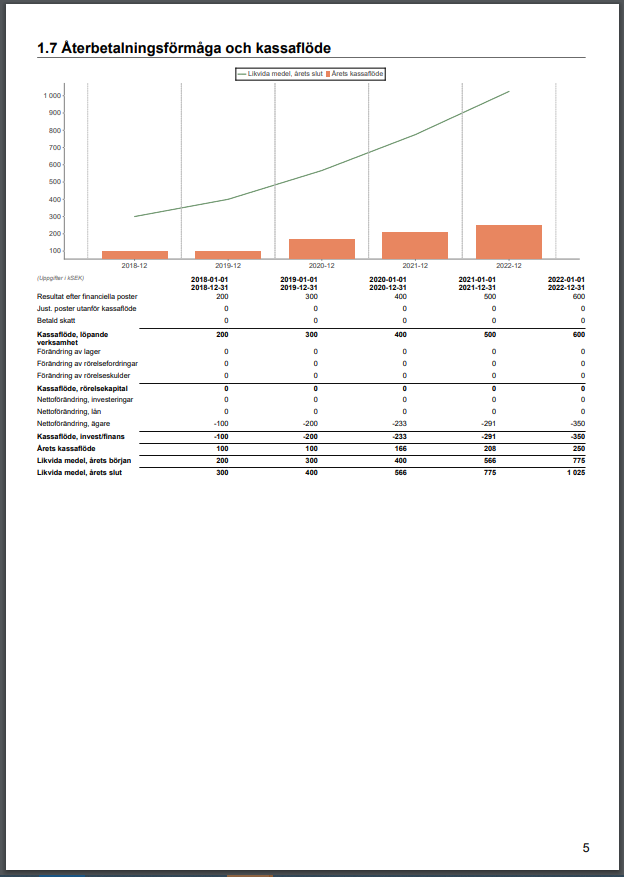
The same call using Java Webservice Client
/* Creates an instance of the webservice client */ ApplicationserviceImpl appImp = new ApplicationserviceImpl(); ApplicationserviceImplSEI app = appImp.getApplicationserviceImplSEIPort(); /* Set endpoint url, username and password (http basic auth) */ Map<String, Object> reqContext = ((BindingProvider) app).getRequestContext(); reqContext.put(BindingProvider.ENDPOINT_ADDRESS_PROPERTY, ENDPOINT); reqContext.put(BindingProvider.USERNAME_PROPERTY, USERNAME); reqContext.put(BindingProvider.PASSWORD_PROPERTY, PASSWORD); //Create the credit case input CompanyCalcInput companyCalcInput1 = new CompanyCalcInput(); companyCalcInput1.setCalculationYear( 2020 ); companyCalcInput1.setCalculationMonth( 10 ); companyCalcInput1.setMainCurrency(Currency.SEK); companyCalcInput1.setCalcInformation( new CalcInformation()); DateWithDay actualDate1 = new DateWithDay(); companyCalcInput1.setActualDate(actualDate1); actualDate1.setDay( 14 ); actualDate1.setYear( 2020 ); actualDate1.setMonth( 10 ); Company company1 = new Company(); companyCalcInput1.getCompanies().add(company1); company1.setID( "5B0A693B-65AB-46B6-826E-7B9362743D4C" ); company1.setCaseSubsidiary(SubsidiaryMode.EXISTING_CASE_SUBSIDIARY); company1.setMainCurrency(Currency.SEK); CompanyInformation companyInformation1 = new CompanyInformation(); company1.setCompanyInformation(companyInformation1); companyInformation1.setOrgNo( "1234567890" ); ActualFigures actualFigures1 = new ActualFigures(); company1.setActualFigures(actualFigures1); actualFigures1.setBalanceSheetCurrentYear( new BalanceSheetPartOfYear()); actualFigures1.setIncomestatementCurrentYear( new IncomestatementPartOfYear()); Incomestatement incomestatement1 = new Incomestatement(); actualFigures1.getHistoricalIncomestatements().add(incomestatement1); incomestatement1.setRevenue( 100000.0 ); Incomestatement incomestatement2 = new Incomestatement(); actualFigures1.getHistoricalIncomestatements().add(incomestatement2); incomestatement2.setRevenue( 200000.0 ); Incomestatement incomestatement3 = new Incomestatement(); actualFigures1.getHistoricalIncomestatements().add(incomestatement3); incomestatement3.setRevenue( 300000.0 ); BalanceSheet balanceSheet1 = new BalanceSheet(); actualFigures1.getHistoricalBalanceSheet().add(balanceSheet1); balanceSheet1.setCash( 100000.0 ); balanceSheet1.setUnrestrictedEquity( 100000.0 ); BalanceSheet balanceSheet2 = new BalanceSheet(); actualFigures1.getHistoricalBalanceSheet().add(balanceSheet2); balanceSheet2.setCash( 200000.0 ); balanceSheet2.setUnrestrictedEquity( 200000.0 ); BalanceSheet balanceSheet3 = new BalanceSheet(); actualFigures1.getHistoricalBalanceSheet().add(balanceSheet3); balanceSheet3.setCash( 300000.0 ); balanceSheet3.setUnrestrictedEquity( 300000.0 ); CreateDocument createDocumentInputPDF = new CreateDocument(); createDocumentInputPDF.setInputData(companyCalcInput1); createDocumentInputPDF.getPrintouts().add( new CreditPMPrintout()); createDocumentInputPDF.setDocumentFormat( "PDF" ); byte [] pdf = app.createDocument(createDocumentInputPDF).getDocument(); //Check the first bytes of the generated pdf Assert.assertEquals( "%PDF-" , new String(pdf).substring( 0 , 5 )); |